Diving into Rust — A Fresh Alternative to Traditional Programming Languages
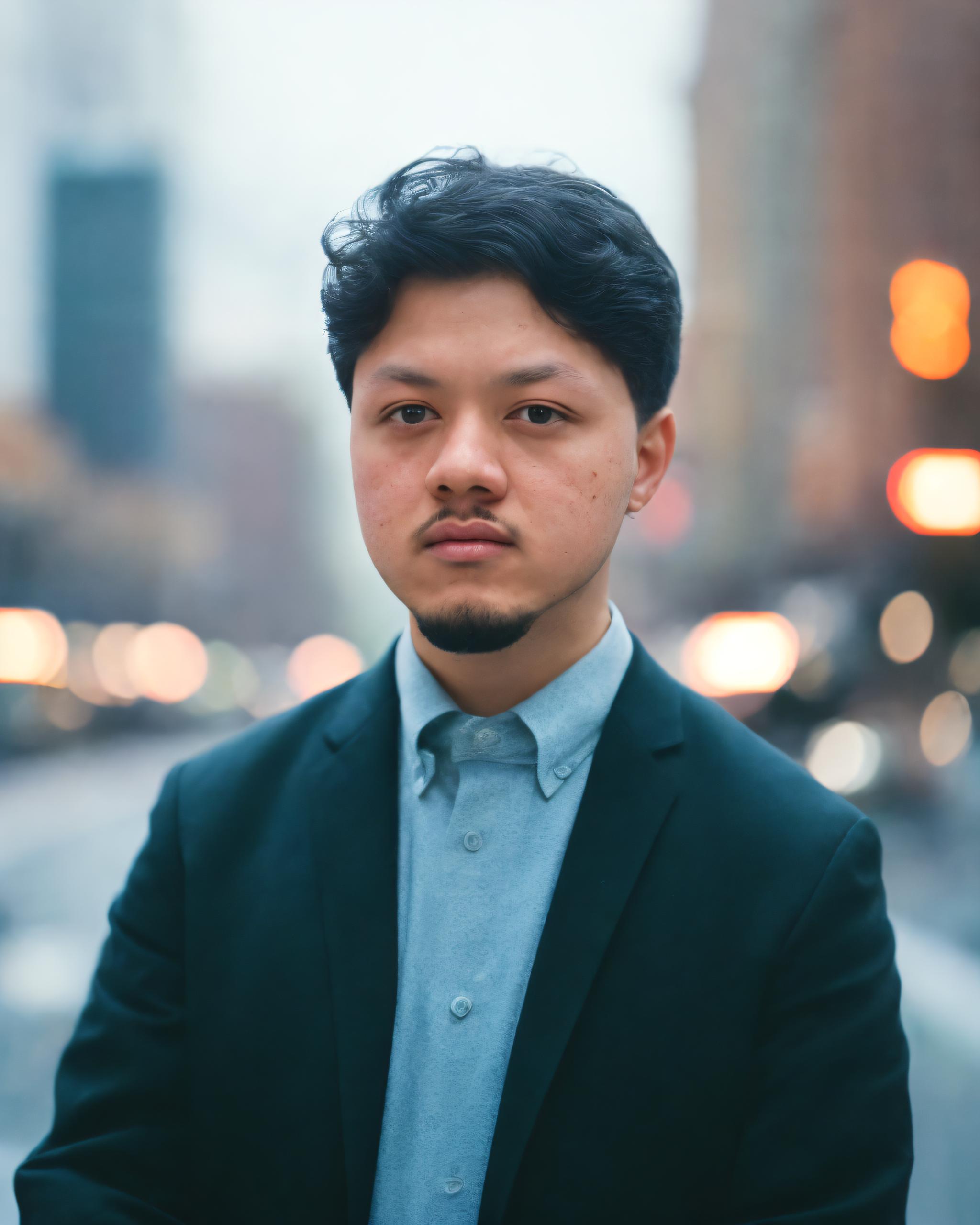
As a passionate developper my goal is to gather a lot of knowledge about programming concepts. And to me that sounded logical to learn high level and low level programming languages.
I really like how Rust is structured and its ecosystem, particuarly Cargo, that’s a simple package and project manager. That’s really simple and the CLI is pretty comfortable to use. Compared to C++, where it’s more complicated to even import a package. We’ve to use alternative as CMake, Mason, Conan, vcpkg, xmake, etc.
I really like the memory safety that I finds really weel compared to C++ as well, the default immutable variable declaration, that suchs a bless ! But also the passing by semantic first in function parameter. There’s no any copy even if there’s some exception for primitive types. We should not get aware for copy and stuff.
There are Option
As an enthusiastic developer committed to broadening my programming acumen, I’ve been diving headfirst into a wide variety of languages, from high-level to low-level. One language that has caught my attention and held it firmly is Rust, and in this article, I aim to share the highlights of my exciting journey with Rust thus far.
Welcoming the Rust Ecosystem
An instant draw for me towards Rust was its package and project manager, Cargo. Rust’s system for handling dependencies is refreshingly simple, and the Cargo CLI offers a user-friendly interface that stands in sharp contrast to the sometimes convoluted procedures in C++.
[package]
name = "my_project"
version = "0.1.0"
authors = ["Your Name <[email protected]>"]
edition = "2018"
[dependencies]
rand = "0.8.4"
In contrast, managing packages in C++ often calls for third-party tools like CMake, Mason, Conan, vcpkg, or xmake, making Cargo’s straightforward approach a breath of fresh air.
The Allure of Memory Safety and Immutability
Rust’s memory safety mechanisms are another aspect I’ve found particularly noteworthy. Rust has safety guards against null reference dereferencing and dangling pointers - a level of protection not always guaranteed in languages like C++.
fn main() {
let mut x = 5;
let r = &mut x;
*r += 1;
println!("x: {}", x);
}
Rust’s design also emphasizes immutability by default, a blessing for any programmer aiming for clean, readable code. In Rust, parameters are semantically passed first into functions, which means no unnecessary copying. This feature, even with a few exceptions for primitive types, takes away the headache of worrying about copy overheads.
Embracing the Option
Rust’s Option
fn divide(numerator: f64, denominator: f64) -> Option<f64> {
if denominator == 0.0 {
None
} else {
Some(numerator / denominator)
}
}
The ease of integrating Option
Semantic Movement: Rust’s Approach to Function Parameters
One aspect of Rust that stands out is its unique approach to handling function parameters. In Rust, the default behavior is to use semantic movement, which essentially means a value is moved into a function when it’s used as a parameter.
fn take_ownership(some_string: String) {
println!("{}", some_string);
}
let s = String::from("hello");
take_ownership(s);
// The variable s is no longer valid here
This mechanism brings significant advantages. Firstly, it’s efficient because there’s no unnecessary copying of data. This becomes more significant with larger data structures. Secondly, it prevents a whole class of bugs. Since the original variable can’t be used after it’s moved, we avoid situations where it might be inadvertently used, causing bugs or crashes.
This contrasts with many other languages, such as C++, where copies are the default behavior when passing parameters to functions. In Rust, however, even though there are exceptions for primitive types, you don’t have to be constantly aware of copy overheads or potential issues related to copying. It’s yet another aspect of Rust that simplifies the programmer’s task while enhancing code safety and performance.
Rust’s Performance Edge
Rust’s performance is impressive. Designed for “bare metal” coding, Rust operates close to the hardware while offering a safer programming environment thanks to its memory management. This combination allows developers to write high-performance applications without fear of crashes due to null or dangling pointers.
fn fibonacci(n: u32) -> u32 {
match n {
0 => 0,
1 => 1,
_ => fibonacci(n - 1) + fibonacci(n - 2),
}
}
The Bottom Line
In essence, my journey into Rust has been a whirlwind of enlightenment and fascination. Rust’s robust set of features, coupled with its emphasis on safety and performance, sets it apart in the programming world. If you’re considering a new language to learn, I wholeheartedly recommend Rust.
Stay tuned as I continue exploring Rust’s myriad features and delve deeper into this captivating language in future posts. Happy coding, everyone!